Date and Time Formatting in Kotlin with the DateTime Library
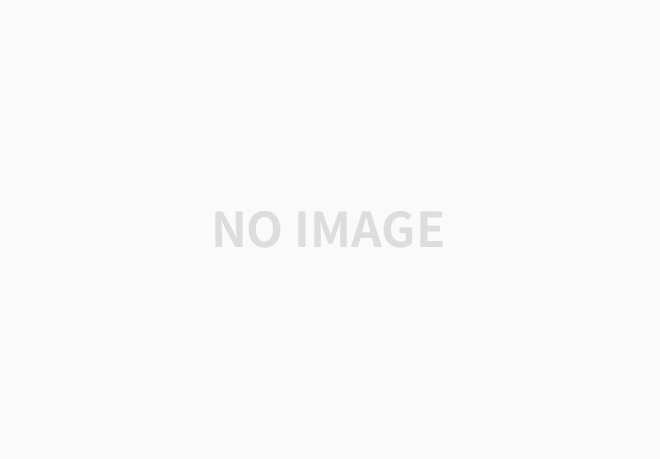
์ต๊ทผ ํ์ฌ ์๋น์ค ๊ฐ๋ฐ ์ค, ์๋ฒ์์ ๋ด๋ ค์ฃผ๋ DateFormat(โ2024-03-11 07:18:25โ)๊ณผ
๋์์ธ์ชฝ์์ ์ํ๋ DateFormat(โ03/11/2024 07:18:25โ)์ด ๋ฌ๋ผ ๋ฐ๋์ ์ ์ฝ์งํ ๊ฒฝํ์ด ์์ด์,,
์ด ์ฃผ์ ๋ฅผ ์ ํํ๊ฒ ๋์์ต๋๋คโฆ๐ฅน
kotlinx-datetime
๋ผ์ด๋ธ๋ฌ๋ฆฌ๋ โv0.6.0-RCโ์์ ์
๋ฐ์ดํธ ๋์์ต๋๋ค.
๊ธฐ์กด์ ๋ ์ง ๋ฐ ์๊ฐ์ ๊ดํ Formatting Options
์๋ฅผ๋ค์ด โMarch 1, 2024, at 9:15 AMโ์ ์ฝ๋๋ก ๋ํ๋ด๊ณ ์ถ๋ค๋ฉด,LocalDateTime(2024, 3, 1, 9, 15, 0, 0)
์ผ๋ก ์์ฑํ ์ ์์ต๋๋ค.
- LocalDateTime
LocalDateTime.Formats.ISO
โ2024-03-01T09:15:00
- LocalDate
LocalDate.Formats.ISO
โ2024-03-01
LocalDate.Formats.ISO_BASIC
โ20240301
- LocalTime
LocalTime.Formats.ISO
โ09:15:00
kotlinx-datetime
๋ผ์ด๋ธ๋ฌ๋ฆฌ๋ฅผ ์ฐ๊ธฐ์ํด์๋build.gradle
๋๋ gradle/libs.versions.toml
์ dependency๋ฅผ ์ถ๊ฐํ๋ฉด ๋ฉ๋๋ค.
implementation "org.jetbrains.kotlinx:kotlinx-datetime:0.6.0-RC.2"
Formatting
๋ ์ง์ ์๊ฐ์ Formatting ํ๋ ๋ฐฉ๋ฒ์ 2๊ฐ์ง๊ฐ ์์ต๋๋ค.
- Unicode Pattern
- Kotlin DSL
์ด์๊ฐ์ ๋ฐฉ๋ฒ์ ์ฌ์ฉํ๋ ค๋ฉด format(...)
ํจ์๋ฅผ ์ฌ์ฉํด์ผ ํฉ๋๋ค.
val currentDateTime = LocalDateTime(2024, 3, 1, 9, 15, 0, 0)
val currentDate = currentDateTime.date
val currentTime = currentDateTime.time
currentDateTime.format(LocalDateTime.Format {
// Unicode Pattern ๋๋ Kotlin DSL
})
currentDate.format(LocalDate.Format {
// Unicode Pattern ๋๋ Kotlin DSL
})
currentTime.format(LocalTime.Format {
// Unicode Pattern ๋๋ Kotlin DSL
})
Unicode Pattern
val currentDateTime = LocalDateTime(2024, 3, 1, 9, 15, 0, 0)
val currentDate = currentDateTime.date
val currentTime = currentDateTime.time
// 2024 03 01 - 09:15
currentDateTime.format(LocalDateTime.Format { byUnicodePattern("yyyy MM dd - HH:mm") })
// 2024/03/01
currentDate.format(LocalDate.Format { byUnicodePattern("yyyy/MM/dd") })
// 09:15
currentTime.format(LocalTime.Format { byUnicodePattern("HH:mm") })
Kotlin DSL
val currentDateTime = LocalDateTime(2024, 3, 1, 9, 15, 0, 0)
val currentDate = currentDateTime.date
val currentTime = currentDateTime.time
// Mar 01 2024 - 09:15
currentDateTime.format(
LocalDateTime.Format {
date(
LocalDate.Format {
monthName(MonthNames.ENGLISH_ABBREVIATED)
char(' ')
dayOfMonth()
chars(" ")
year()
}
)
chars(" - ")
time(
LocalTime.Format {
hour(); char(':'); minute()
}
)
}
)
// Mar 01, 2024
currentDate.format(LocalDate.Format {
monthName(MonthNames.ENGLISH_ABBREVIATED)
char(' ')
dayOfMonth()
chars(", ")
year()
})
// 09:15
currentTime.format(LocalTime.Format {
hour()
char(':')
minute()
})
hour()
ํจ์๋ 0์์ 23์๊น์ง ์๊ฐ์ ๋ฆฌํดํฉ๋๋ค.- ๋ง์ฝ 12์๊ฐ์ ๋ก ์๊ฐ์ ํ์ํ๋ ค๋ฉด
amPmHour()
ํจ์๋ฅผ ์ฌ์ฉํด์ผ ํฉ๋๋ค.
Kotlin DSL ๋ฐฉ์์ ์ฌ์ฉํ๋ฉด, padding
๋งค๊ฐ๋ณ์๋ก year()
monthNumber()
dayOfMonth()
hour()
minute()
์ ๊ฐ์ ํจ์๋ค์ ์ ๋ฌํ ์ ์๊ณ , ์ด๋ฅผ ํตํด ํจ๋ฉ ์คํ์ผ์ ๋ณ๊ฒฝํ ์ ์์ต๋๋ค.
val time = LocalTime(hour = 9, minute = 8)
// "9:8"
time.format(LocalTime.Format {
hour(padding = Padding.NONE)
char(':')
minute(padding = Padding.NONE)
})
// "09:08"
time.format(LocalTime.Format {
hour(padding = Padding.ZERO)
char(':')
minute(padding = Padding.ZERO)
})
// " 9: 8"
time.format(LocalTime.Format {
hour(padding = Padding.SPACE)
char(':')
minute(padding = Padding.SPACE)
})
Parsing
Formatting๋ ๋ ์ง์ ์๊ฐ์ parse
ํจ์๋ฅผ ์ฌ์ฉํ ์ ์์ต๋๋ค.
val formattedDateTime = "2024 03 01 - 09:15"
LocalDateTime.parse(
input = formattedDateTime,
format = LocalDateTime.Format { byUnicodePattern("yyyy MM dd - HH:mm") }
)
val formattedDate = "2024/03/01"
LocalDate.parse(
input = formattedDate,
format = LocalDate.Format { byUnicodePattern("yyyy/MM/dd") }
)
val formattedTime = "09:15"
LocalTime.parse(
input = formattedTime,
format = LocalTime.Formats.ISO
)
์ฆ, Unicode Pattern ๋๋ Kotlin DSL๋ก ๋ ์ง์ ์๊ฐ์ Formatting โ parse
ํจ์๋ก ์ ์ ํ ๊ณณ์ ์ฌ์ฉ!
Reference
https://alexzh.com/date-and-time-formatting-in-kotlin-with-the-datetime-library/
Date and Time Formatting in Kotlin with the DateTime Library
Explore the art of formatting date and time in Kotlin with the Kotlinx-datetime library. This guide introduces you to the efficient use of Unicode patterns and Kotlin DSL for formatting date and time types. Ideal for Kotlin Multiplatform projects.
alexzh.com